Some things to consider as a software engineer... that have nothing to do with writing code
A non-exhaustive list that I'm writing for myself on the important soft skills I've had to cope with working professionally in tech. I started writing it in Obsidian as a personal note and thought why not share in case it can help someone like me down the road.
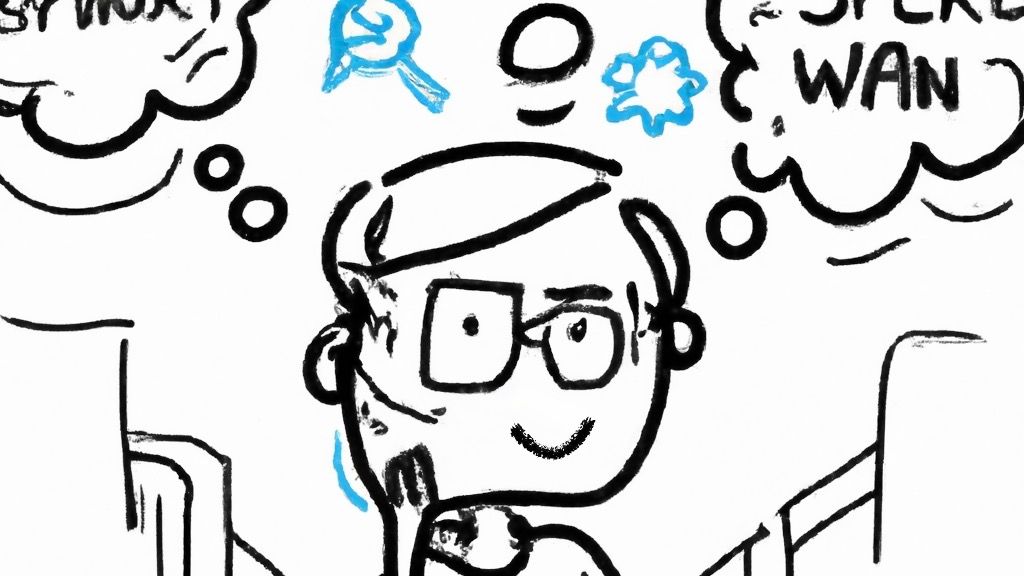
Keep in mind these are just my current opinions in the context of building a production-ready app in your professional career.
Disclaimer: some of these are a bummer as curious minds that want to work with the latest tech and cool projects involving going to mars or building robots (or space traveling robots). There not meant to be a bummer, but more of a thing to consider in the back of your head when you feel stuck on a problem or are starting something new and have a lot of decisions to make.
Only engineers care about tech
The people using your app will not care that you used the new SvelteKit release with real-time GraphQL subscriptions, and a brand new backend refactored to use Rust and is 100% serverless.
Buzzwords and cool tech is AWESOME. It's probably the thing that I get excited about the most often. And as a frontend engineer, the landscape that I work with is constantly changing to newer and better things. Don't ignore these things. But keep them as side projects until they're stable and you know you and your team can work fast using them at your gig.
Keep it simple stupid (KISS) is a real challenge on greenfield projects
Similar to the first point on wanting to use cool tech, I naturally find myself wanting to build big and exciting things. Especially the more senior that I get. Writing CRUD for the nth time isn't as exciting anymore. I get it.
Go with the most bare bones approach that you can to get the thing out the door and validate that it's what you actually want or need to build. Then the challenge is to avoid worrying about things like scale or performance too much unless they're a problem.
The right tech isn't always the best tech
Quick example: you have a requirement for "high performance". Boom - let's use Rust!
That's probably not the best answer unless:
- You and your team know rust well
- Most of what you're solving is actively talked about and worked on in the community
- You've already built a handful of completed projects running in production written in Rust and you're happy with the tradeoffs
This isn't specific to Rust at all, but the idea that you choose what tech to build with purely based on it fitting the problem. People challenges are usually harder than tech challenges and you can't ignore what's best for the people building your app.
Also keep in mind that your requirements probably aren't reliant on tech much. Like the "high performance" requirement we mentioned earlier... What do you mean by "high performance?" How many milliseconds are we talking? What type of data is it - static, dynamic? Can we cache the results using a CDN and proper cache headers, or Redis? You get the point. There are plenty of ways to accomplish the same goal and most of the most impactful solutions are not choosing a specific tech.
Focus on things that won't change in X years
Things are always changing. Especially in tech. And even more so in frontend tech. You could spend all of your weekends mastering every detail of X framework or new trendy library. And that could be a huge benefit for you for the next 10 years if it's really popular. But it'll probably get shunned out eventually by the next cool thing and be somewhat obsolete.
The things that won't change though are writing code that is easy to follow, documenting your architecture and decisions well, automating tests that follow the way users will interact with your app, etc.
Keep your scope small
Projects that drag on are projects that don't get finished. You have a finite amount of novelty inspiration when starting a new prject that you have to protect. It's all too easy to bail when you get too in the weeds and feel like the project is way more complex than you thought.
Small scope and many small wins consistently > large scope and bigger wins less often
As we've mentioned earlier, requirements will change too. Which sucks when you're halfway through a huge project and have to refactor a lot of files. Keeping things small makes you more limber and nimble when having to pivot. Which will almost inevitably happen.
Avoid committing to things you can't control
Most of the things you'll build aren't the same thing you've built before - if they were, that'd be boring and wouldn't grow you in your career either, but that's a different problem.
With that said, how can you be sure of how long it'll take you to do something that you've never done before? You're not a factory making another bag of potato chips, just like a million others. You're building something with unique requirements to the business, with different managers, developers, and goals than any other project out there. Even if it's only different by a nuanced detail.
It's unreasonable to expect for a business or manager to be ok with saying "IDK how long it'll take" though too. They have to plan around the development, ensure it's the right thing to focus on based on cost/impact and is the best for them and their customers. So we need to estimate things, but how?
I don't have a silver bullet answer, and if you do, I probably won't believe you. But a few general heuristics that help me are:
- If you can triple the amount of time you think it'll take, and that's still a huge value add that's more impactful than something else. It's probably a good idea to go for it.
- Communicate early and often on risks, challenges and milestones to ensure your leadership is aware of the things you're running into and can plan around them.
- Document your process and learnings as you go. This will help you and others down the road as you go down the same rabbit hole of questions and forget why you ended up choosing the route you're on.
- The last point deserves its own section. See "Stakeholders don't know what they want"
Stakeholders don't know what they want
Validate what you're building with the stakeholders as early and often and you can. There's almost nothing worse than spending a lot of time and effort on something that no one ever uses or wants.
The only way you'll ever really know is if people actually use it. And they'll never know what they really need until they have it in their hands to use themselves.
Bonus points if you're building something you can benefit from yourself. Then you can "dogfood" the product you're building and understand all of its quirks and pain points like your users will.
Communication and finding answers is the most important skill you have
You can't hold everything you learn in your head. And if you can, I probably won't believe you. Looking things up is one of the most valuable things you can focus your time on mastering. Focus on using search engines, knowing where to look for the right thing and filtering out the nonsense.
For example, if there's an error on your production app, where should you look first? The logs, a search engine, an internal wiki, etc.
Some common generalizations that may be helpful:
- Generic web problem or question, search
<my query> mdn
to see what answers are available on MDN (Mozilla Web Docs). - Framework specific web problem, search
<my query> framework
- Looking for a package,
<my query> <package-manager>
, i.e.state machine npm
- Looking for tribal knowledge from your team, write a message in your chat with what you've already tried, what you're trying to solve (not just how to do X) and where you've already looked and searched for. You're much more likely to find answers that way.
All decisions have tradeoffs
There are no silver bullets. Everything you do will be a shit sandwich.
Bleeding edge technology? Painful growing pains that haven't been solved yet. Possibly some bugs. Lots of heartache. But probably some really nice benefit.
Old school, tried and true technology? Probably great support in the community, lots of documentation, minimal bugs. But doing something modern might be clunkier than the latest and greatest, and you may not be able to take advantage of the newest performance and features that a modern approach offers.
Everything you work on is one if statement away from crashing
Most things feel duck taped together. No matter how large your scale is, how smart your colleagues are or how much money your product is making. It's not always a bad thing, unless you're terrified to break things every time you make a change or your user's data is at risk.
This is why automated and manual testing is so valuable. Especially using the product yourself all the time if possible. the faster you find the edge cases, limitations and bugs, the better. it's always better to find and fix things before your users do.
Always be cautious when touching production code for this reason. Especially risky things are find and replace, removing functionality that spans across the application, or touching areas that aren't well understood or tested. Double your caution and testing on these changes.
Avoid judging those who wrote code before you
Related to the last point, the code you're working on has probably made the company money and provided value. If it hasn't, it probably was something that needed to be validated by building it first and it was the right thing to do. Maybe not, but you only know what you know and just because you have hindsight now, always remember that the information you have now likely wasn't available at the time this was being built.
I'm also pretty sure that you hate code that you wrote yourself. Even if just from a few weeks ago! Generally speaking, everyone is constantly learning, doing the best they can with the information they have at the time. This is where documentation on architecture design and decisions is so important. You only know what you know right now and waiting too long is almost always worse than starting something now. This last statement is arguable, but especially in a startup world, moving fast is sometimes even more important than the quality of the product. At least in the beginning.
To do something right, it helps to build it 3 times
Rather than thinking through the perfect way to do something for a month, I'd argue that spending a month rebuilding the feature over and over is more valuable time spent. It reminds me of the anecdotal story of the teacher that told half of the class to get a grade was to make as many clay pots as possible. The other half was to make the best quality pots they could. Skip to the end and the students that had the best quality pots were the ones that made the most. By a long shot.
Don't rush into things just to follow some anecdotal story, but don't spend a ton of time diving too deep into the weeds without actually just getting your hands dirty.
Conclusion
Hope you enjoyed! I'll probably have more posts like this as the years go by and I learn more. But for now, these informal tenets help guide me from 9-5. Enjoy, and happy coding. SL